Sphinx RST Cheat Sheet for Python Docstrings
Inline Markup
*italics*
is one asterisk**bold**
is two asterisks``code``
is in backticksbold is two asterisks
code
is in backticks
Links
`<www.python.org>`_
`python <www.python.org>`_
Internal Links
.. _link_name:
(Note the blank line)
Named links can be referenced with:
:ref:`link_name`
or
:ref:`alternate text <link_name>`
RST documentation says you can link implicitly using title names, but this doesn't work across files. Always use the ":ref:" mechanism.
Lists
* Item one
* Item two with a
longer line (note indentation)
1. Numbered
2. Works
#. As well
#. Notice a single blank line doesn't create a new list
- Item one
- Item two with a longer line (note indentation)
- Numbered
- Works
- As well
- Notice a single blank line doesn't create a new list
Code
Code is displayed with a formatting directive which Sphinx will then highlight using pygments.
.. code-block:: python
:linenos:
for x in range(1, 10):
print(x)
1 2 | for x in range(1, 10): print(x) |
Notice the blank line between the directive and the indented content
Including Files
You can include parts of a file.
.. include:: filename
:start-line: 3
:end-line: 3
:start-after: 3
:end-before: 5
Verbatim include is done using a different directive.
.. literalinclude:: filename
:linenos:
:language: python
:lines: 1, 3-5
:start-line: 3
:end-line: 3
:start-after: 3
:end-before: 5
References in Code
Within a docstring you can reference modules, classes and functions.
""" |
Links to a class in the same file Links to a function in the same file Displays an attribute name, no link |
:mod:`full.module.name`
|
Links to another module |
:class:`module.ElsewhereClass` |
Links to a class in a different file Links to a function in a different file |
A name can be prefixed with "." to search for a match within the module. It can also be prefixed with "~" to have the resulting link be the short-form version of a module. These can be used together. An alternate name can be used by specifying both the title and a target, wrapping the target in angle brackets.
""" |
Looks for class somewhere in the module Fully qualified class but only shows "ElsewhereClass" in the link Looks for class and only shows relative name Links to "SomeClass" but displays "Some class" in the text |
Headings
Heading depth is determined based on context, but being consistent is helpful. Typical choices are:
*****
Title
*****
#########
Sub-Title
#########
Section
=======
Sub-Section
-----------
Title
Sub-Title
Section
Sub-Section
Sub-Title
Section
Sub-Section
Section
Sub-Section
Sub-Section
Horizontal Rule
A horizontal separator can be created using any puntation in a row repeated more than 4 times.
------------
Images
.. image:: monet_wildenstein.png
:width: 309px
:align: left
:height: 208px
:alt: Monet Wildenstein
.. figure:: monet_waterloo_bridge.jpg
:figclass: align-center
Figures are like images with a caption
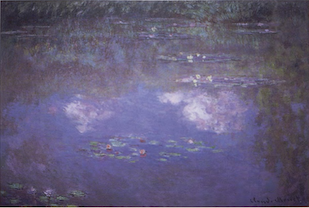
Figures are like images with a caption
Notes & Warnings
.. note:: This is a **note** box
.. warning:: Note the space between directive and the text
- Note
- This is a note box
- Warning
- Note the space between the directive and the text
Sphinx Autodoc Extension
Using the autodoc extension you can include the pydoc from your program.
.. automodule:: module.name |
Include functions that start with "_" and "__" Include functions that start and end with "__". Include members without pydoc Members to exclude Order of included classes One of: alphabetical (default), groupwise (by type), or bysource |
Both members
and special-members
can have no
parameters to include all applicable information.
Unless special-members
is included, __init__
is left out. The class description does include the paramters from the
constructor though, so it is best practice to put the parameter
descriptions for the constructor in the class description. Example:
class Foo:
""The foo class does stuff and
things.
:param bar: This is the bar parameter to
the Foo constructor
"""
def __init__(self, bar):
# no pydoc
here!
pass
Pieces of Code
Instead of documenting the module you can specify a class or function.
.. autoclass:: module.ClassName
:members:
or
.. autofunction::
.. autodecorator::
.. autodata::
.. automethod::
.. autoattribute::
Sphinx Conf
conf.py
to use some variables:
# -- Project information ----------------------------------------------------- project = 'My Project' copyright_start = 2019 author = 'Arthur Author' import datetime year = datetime.datetime.now().year if year == copyright_start: copyright = '%d, %s' % (year, author) else: copyright = '%d-%d, %s' % (copyright_start, year, author) # short version number (X.Y) import imp mod = imp.load_source('project_mod', '../project/__init__.py') version = mod.__version__
Sphinx Conf and Django
CUR_DIR = os.path.join(os.path.dirname(__file__)) PRJ_DIR = os.path.abspath(os.path.join(CUR_DIR, 'awl')) sys.path.insert(0, os.path.abspath('..')) import django from django.conf import settings settings.configure( BASE_DIR=PRJ_DIR, INSTALLED_APPS=( 'django.contrib.auth', 'django.contrib.contenttypes', 'django.contrib.admin', 'my_project', ), ) django.setup()